728x90
import matplotlib.pyplot as plt
import numpy as np
figsize = (7, 7)
In [ ]:
import matplotlib.pyplot as plt
fig, ax = plt.subplots(figsize=figsize)
ax.scatter(0, 0,
s=10000,
facecolor='red',
edgecolor='b',
linewidth=5)
Out[ ]:
<matplotlib.collections.PathCollection at 0x16be1ea00>
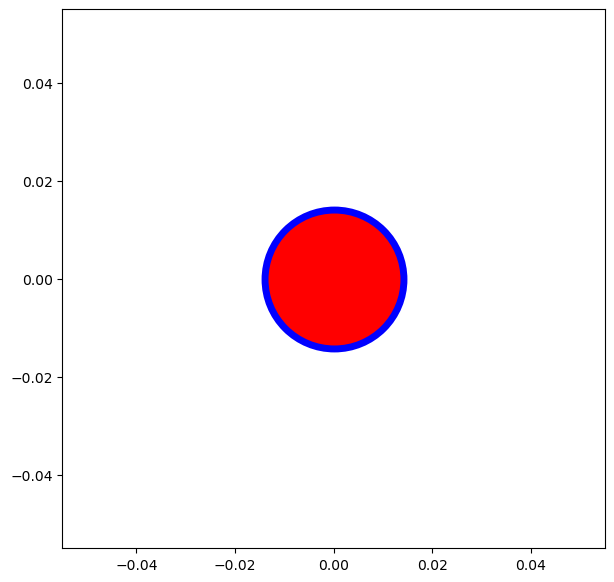
In [ ]:
fig, ax = plt.subplots(figsize=figsize)
n_data = 100
x_data = np.random.normal(0, 1, (n_data,))
y_data = np.random.normal(0, 1, (n_data,))
ax.scatter(x_data, y_data,
s=100,
facecolor='white', # 중첩 확인 불가
edgecolor='tab:blue',
linewidth=1)
Out[ ]:
<matplotlib.collections.PathCollection at 0x16bd01f10>
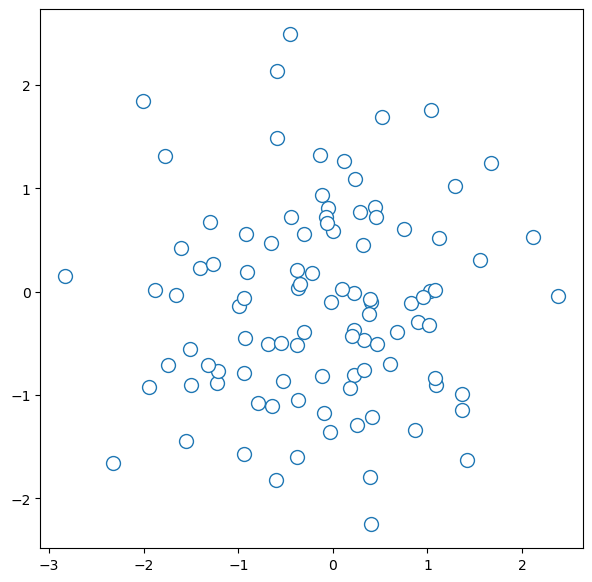
In [ ]:
fig, ax = plt.subplots(figsize=figsize)
n_data = 100
x_data = np.random.normal(0, 1, (n_data,))
y_data = np.random.normal(0, 1, (n_data,))
ax.scatter(x_data, y_data,
s=100,
facecolor='None',# 중첩 확인 가능
edgecolor='tab:blue',
linewidth=1)
Out[ ]:
<matplotlib.collections.PathCollection at 0x16bca3190>
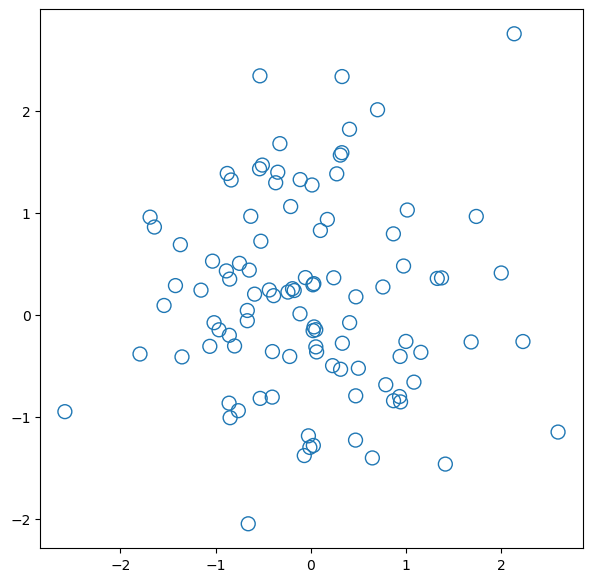
In [ ]:
fig, ax = plt.subplots(figsize=figsize)
n_data = 100
x_data = np.random.normal(0, 1, (n_data,))
y_data = np.random.normal(0, 1, (n_data,))
ax.scatter(x_data, y_data,
s=100,
facecolor='None',
edgecolor='tab:blue',
linewidth=2,
alpha=0.5) # 투명도
plt.show()
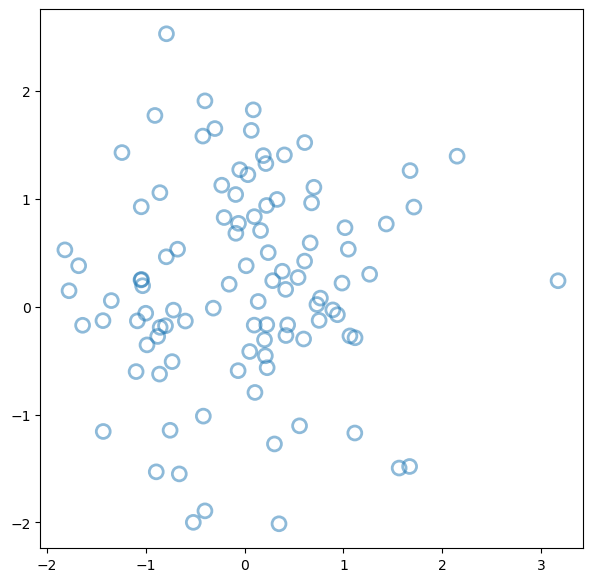
In [ ]:
n_data = 100
s_idx = 30
x_data = np.arange(s_idx, s_idx + n_data)
y_data = np.random.normal(0, 1, (n_data,))
fig, ax = plt.subplots(figsize=figsize)
ax.plot(x_data, y_data)
fig.tight_layout(pad=1) #pad : Padding between the figure edge and the edges of subplots. 여백
x_ticks = \
np.arange(s_idx, s_idx + n_data + 1, step=29)
ax.set_xticks(x_ticks)
ax.tick_params(labelsize=15)
ax.grid()
plt.show()
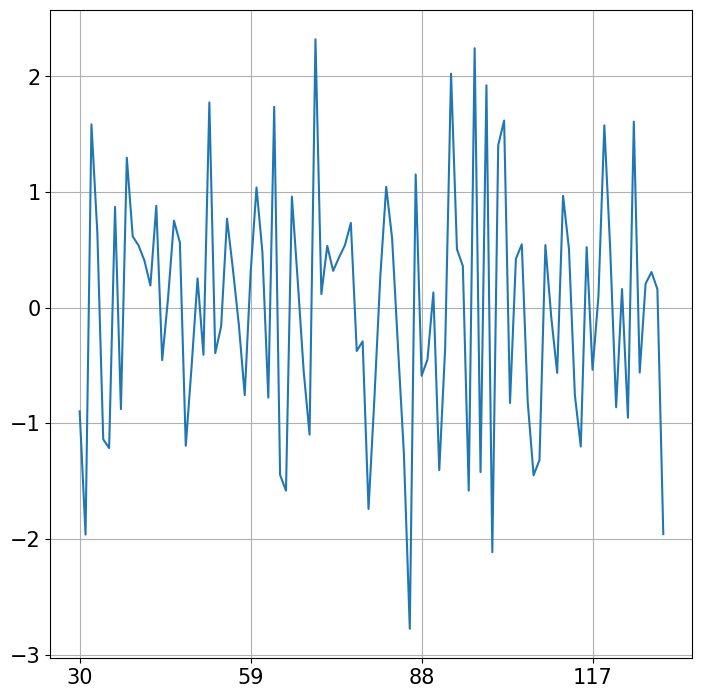
In [ ]:
np.random.seed(404)
x_data = np.array([10, 25, 31, 40, 55, 80, 100])
y_data = np.random.normal(0, 1, (7, ))
fig, ax = plt.subplots(figsize=figsize)
ax.plot(x_data, y_data)
fig.subplots_adjust(left=0.2)
ax.tick_params(labelsize=10)
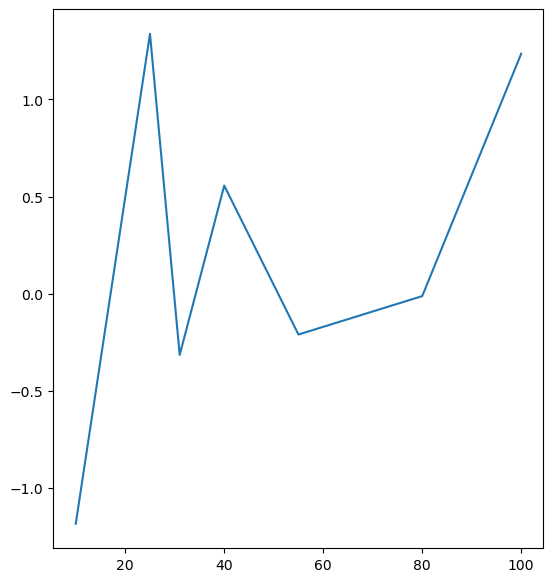
In [ ]:
ax.set_xticks(x_data)
ylim = ax.get_ylim() # The current y-axis limits in data coordinates.
# ylim에 y 데이터 좌표 limits 추출. 다시 num=8(개) 로 yticks 생성. set_yticks 로 재설정
yticks = np.linspace(ylim[0], ylim[1], 8)
ax.set_yticks(yticks)
ax.grid()
plt.show()
In [ ]:
PI = np.pi
t = np.linspace(-4*PI, 4*PI, 300)
sin = np.sin(t)
linear = 0.1*t
fig, ax = plt.subplots(figsize=figsize)
ax.plot(t, sin)
ax.plot(t, linear)
ax.set_ylim(-1.5, 1.5)
x_ticks = np.arange(-4*PI, 4*PI+0.1, PI)
x_ticklabels = [str(i) + r'$\pi$' for i in range(-4, 5)]
ax.set_xticks(x_ticks)
ax.set_xticklabels(x_ticklabels)
ax.tick_params(labelsize=10)
ax.grid()
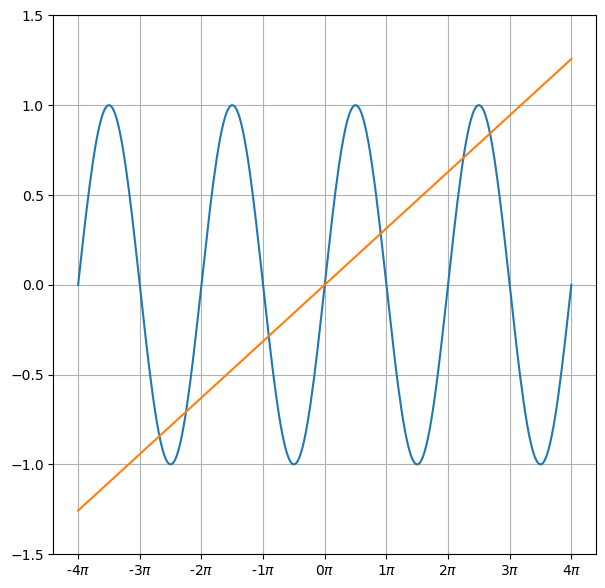
In [ ]:
PI = np.pi
t = np.linspace(-4*PI, 4*PI, 1000)
sin = np.sin(t)
cos = np.cos(t)
tan = np.tan(t)
fig, axes = plt.subplots(3, 1, figsize = figsize)
axes[0].plot(t, sin)
axes[1].plot(t, cos)
# axes[2].plot(t, tan)
# axes[2].set_ylim(-5, 5)
# tan 발산하는 그래프이다.
# 트릭을 사용하여 정상적으로 그리자
# y[:-1][np.diff(y) < 0] = np.nan # np.nan means NA. 값을 없애버린다.
tan[:-1][np.diff(tan) < 0] = np.nan
axes[2].plot(t, tan)
axes[2].set_ylim(-5, 5)
# grid()
for ax in axes:
ax.grid()
fig.tight_layout()
In [ ]:
PI = np.pi
t = np.linspace(-4*PI, 4*PI, 1000).reshape(1, -1)
# 이번 예습은 reshape 안해도 결과가 나온다. 그래도 연습한다.
# reshape 의 -1은 컴터가 알아서 찾아준다.
# reshape(1, -1) : (1000, ) -> (1, 1000)
# reshape(-1, 1) : (1000, ) -> (1000, 1)
sin = np.sin(t) # shape -> (1, 1000)
cos = np.cos(t) # shape -> (1, 1000)
tan = np.tan(t) # shape -> (1, 1000)
# tan[:-1][np.diff(tan) < 0] = np.nan
data = np.vstack((sin, cos, tan)) # shape -> (3, 1000)
title_list = [r'$sin(t)$', r'$cos(t)$', r'$tan(t)$']
x_ticks = np.arange(-4*PI, 4*PI+PI, PI)
x_ticklabels = [str(i) + r'$\pi$' for i in range(-4, 5)]
fig, axes = plt.subplots(3, 1,
figsize=figsize,
sharex=True)
# for ax_idx, ax in enumerate(axes.flat):
for ax_idx, ax in enumerate(axes):
ax.plot(t.flatten(), data[ax_idx])
ax.set_title(title_list[ax_idx], fontsize=10)
ax.tick_params(labelsize=10)
ax.grid()
if ax_idx == 2:
ax.set_ylim([-3, 3])
fig.subplots_adjust(left=0.1, right=0.95,
bottom=0.05, top=0.95)
axes[-1].set_xticks(x_ticks)
axes[-1].set_xticklabels(x_ticklabels)
Out[ ]:
[Text(-12.566370614359172, 0, '-4$\\pi$'),
Text(-9.42477796076938, 0, '-3$\\pi$'),
Text(-6.283185307179586, 0, '-2$\\pi$'),
Text(-3.141592653589793, 0, '-1$\\pi$'),
Text(0.0, 0, '0$\\pi$'),
Text(3.141592653589793, 0, '1$\\pi$'),
Text(6.283185307179586, 0, '2$\\pi$'),
Text(9.42477796076938, 0, '3$\\pi$'),
Text(12.566370614359172, 0, '4$\\pi$')]
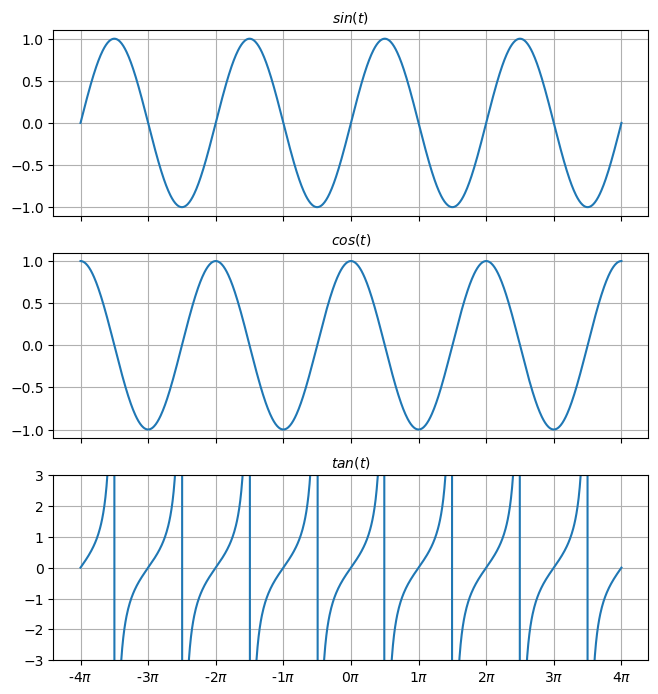
In [ ]:
# 점근선
fig, ax = plt.subplots(figsize=figsize)
ax.set_xlim(-5, 5)
ax.set_ylim(-5, 5)
ax.axvline(x=1,
color='black',
linewidth=1)
Out[ ]:
<matplotlib.lines.Line2D at 0x16c6124f0>
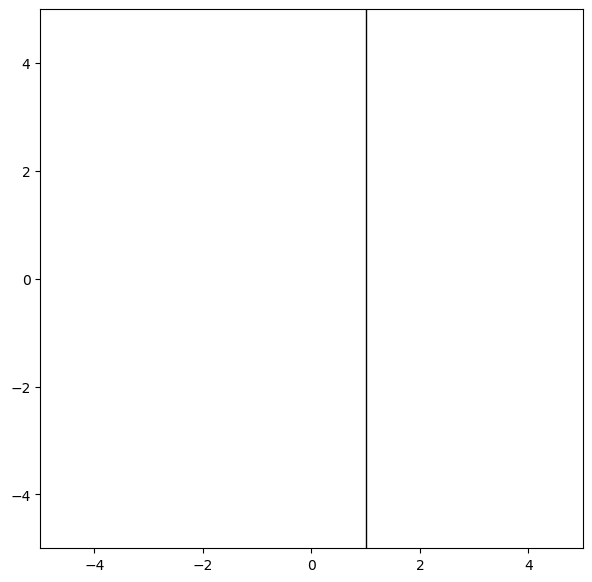
In [ ]:
# 점근선
fig, ax = plt.subplots(figsize=figsize)
ax.set_xlim(-5, 5)
ax.set_ylim(-5, 5)
ax.axvline(x=1,
ymax=0.8, ymin=0.2,
color='black',
linewidth=1)
Out[ ]:
<matplotlib.lines.Line2D at 0x16bd0a3d0>
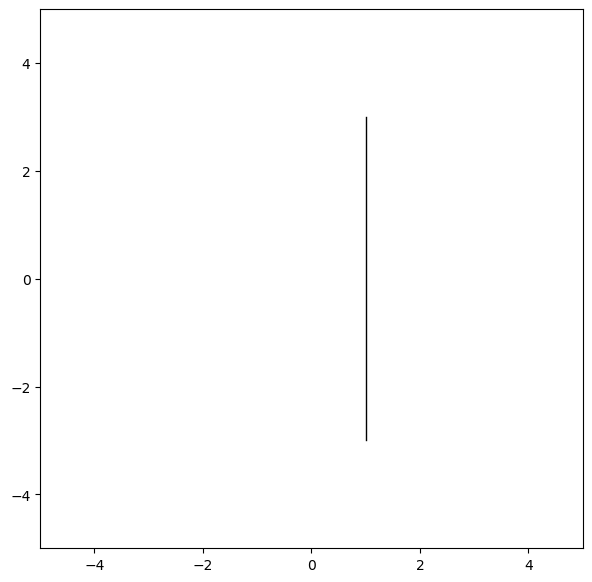
In [ ]:
x = np.linspace(-4*np.pi, 4*np.pi, 200)
sin = np.sin(x)
fig, ax = plt.subplots(figsize=figsize)
ax.plot(x, sin)
ax.axhline(1, ls=':', lw=1, color='gray')
ax.axhline(-1, ls=':', lw=1, color='gray')
Out[ ]:
<matplotlib.lines.Line2D at 0x16c0b8dc0>
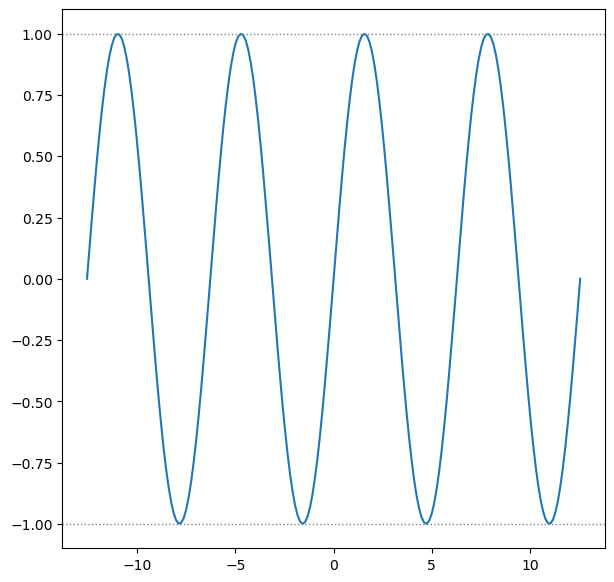
In [ ]:
np.random.seed(0)
n_data = 100
random_noise1 = np.random.normal(0, 1, (n_data, ))
random_noise2 = np.random.normal(1, 1, (n_data, ))
random_noise3 = np.random.normal(2, 1, (n_data, ))
fig, ax = plt.subplots(figsize=figsize)
ax.tick_params(labelsize=10)
ax.plot(random_noise1, label='random noise1')
ax.plot(random_noise2, label='random noise2')
ax.plot(random_noise3, label='random noise3')
# ax.legend(fontsize=5, loc='upper right')
ax.legend(fontsize=5, loc='center right')
Out[ ]:
<matplotlib.legend.Legend at 0x16c432400>
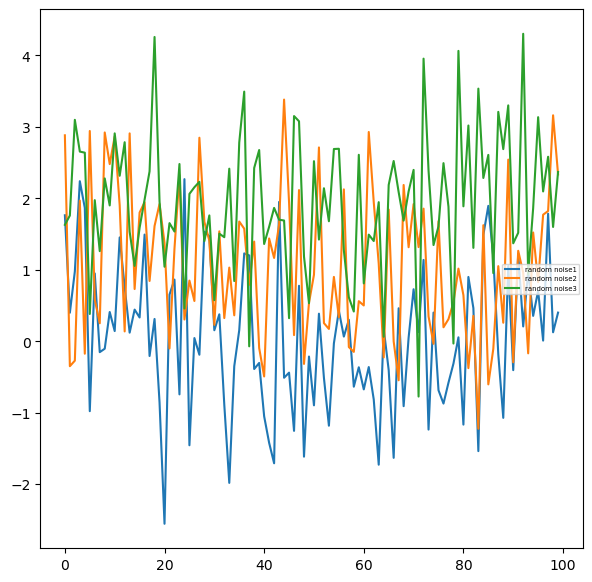
In [ ]:
# bbox_to_anchor
# If "loc", update the loc parameter of the legend upon finalizing.
# If "bbox", update the bbox_to_anchor parameter. loc 보다 bbox 선적용 된다.
# bbox의 기준에서 loc가 적용된다.
np.random.seed(0)
n_data = 100
random_noise1 = np.random.normal(0, 1, (n_data, ))
random_noise2 = np.random.normal(1, 1, (n_data, ))
random_noise3 = np.random.normal(2, 1, (n_data, ))
fig, ax = plt.subplots(figsize=figsize)
ax.tick_params(labelsize=10)
ax.plot(random_noise1, label='random noise1')
ax.plot(random_noise2, label='random noise2')
ax.plot(random_noise3, label='random noise3')
ax.legend(fontsize=8, bbox_to_anchor=(1, 0.5) ,loc='center left')
fig.tight_layout()
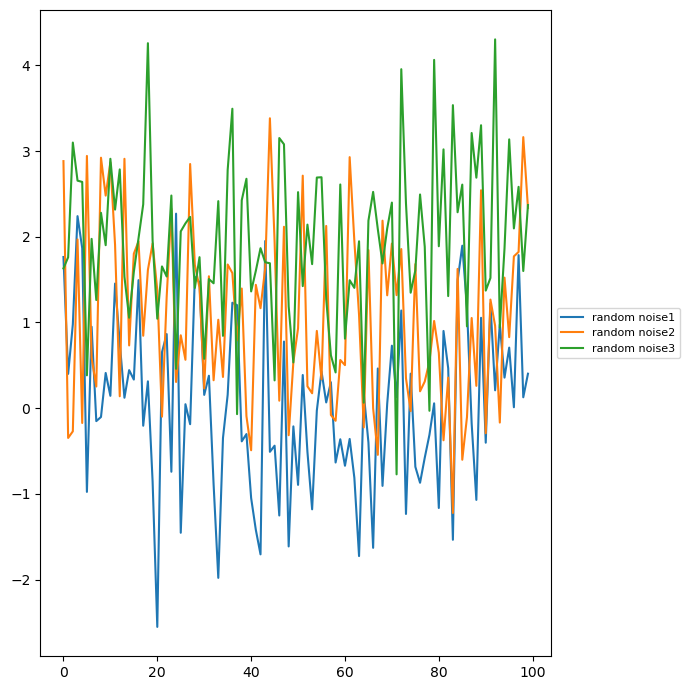
In [ ]:
PI = np.pi
t = np.linspace(-4*PI, 4*PI, 300)
sin = np.sin(t)
fig, ax = plt.subplots(figsize=figsize)
for ax_idx in range(12):
label_template = 'added by {}' # == f'added by {ax_idx}'
ax.plot(t, sin+ax_idx,
label=label_template.format(ax_idx))
ax.legend(fontsize=7,
ncol=4,
bbox_to_anchor=(0.5, -0.1),
loc='upper center')
fig.tight_layout()
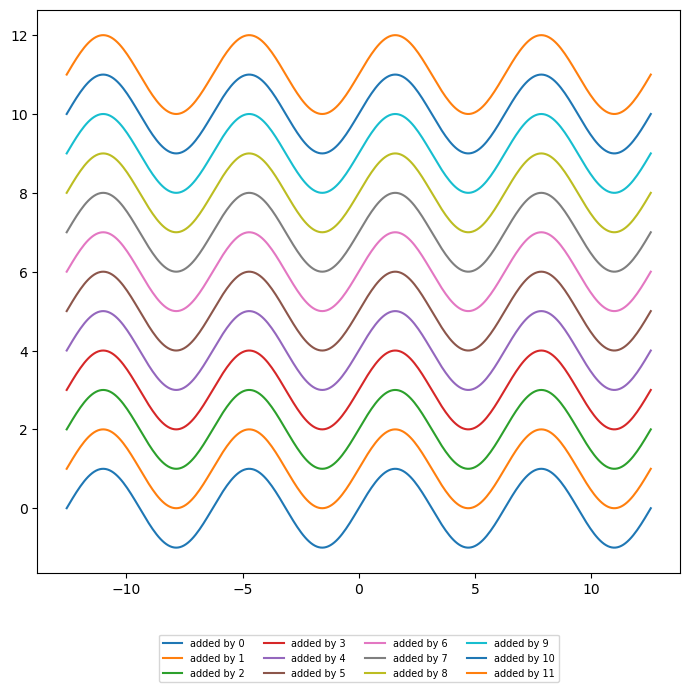
In [ ]:
np.random.seed(0)
fig, ax = plt.subplots(figsize=figsize)
data1 = np.random.normal(0, 1, 100)
data2 = np.random.normal(5, 2, 200)
data3 = np.random.normal(13, 3, 300)
xticks = np.arange(3)
violin = ax.violinplot([data1, data2, data3],
showmeans=True, # 평균. 바이올린의 중간에 표시
quantiles=[[0.25, 0.75], [0.1, 0.9], [0.3, 0.7]], # 지점 표시
positions=xticks)
ax.set_xticks(xticks)
ax.set_xticklabels(['setosa', 'versicolor', 'virginica'])
ax.set_xlabel('Species', fontsize=10)
ax.set_ylabel('Values', fontsize=10)
print(violin.keys()) # dict_keys(['bodies', 'cmeans', 'cmaxes', 'cmins', 'cbars', 'cquantiles'])
violin['bodies'][0].set_facecolor('blue')
violin['bodies'][1].set_facecolor('red')
violin['bodies'][2].set_facecolor('green')
violin['cbars'].set_edgecolor('gray')
violin['cmaxes'].set_edgecolor('gray')
violin['cmins'].set_edgecolor('gray')
violin['cmeans'].set_edgecolor('gray')
plt.show()
dict_keys(['bodies', 'cmeans', 'cmaxes', 'cmins', 'cbars', 'cquantiles'])
<matplotlib.collections.PolyCollection object at 0x16c1c8c40>
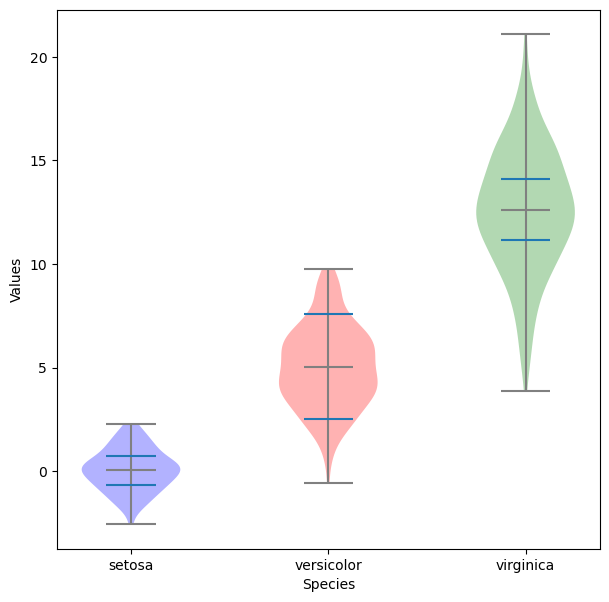
728x90
'새싹 > TIL' 카테고리의 다른 글
[핀테커스] 231006 matplot - iris (0) | 2023.10.06 |
---|---|
[핀테커스] 231005 matplot 실습 (0) | 2023.10.05 |
[핀테커스] 230922 python 수학 실습 (0) | 2023.09.22 |
[핀테커스] 230921 python 수학 실습 (1) | 2023.09.21 |
[핀테커스] 230920 python 수학 실습 (0) | 2023.09.20 |