import matplotlib.pyplot as plt
import numpy as np
In [ ]:
fig = plt.figure(figsize=(7, 7),
facecolor='linen')
ax = fig.add_subplot()
ax.plot([2, 3, 1])
ax.scatter([2, 3, 1], [2, 3, 4])
plt.show()
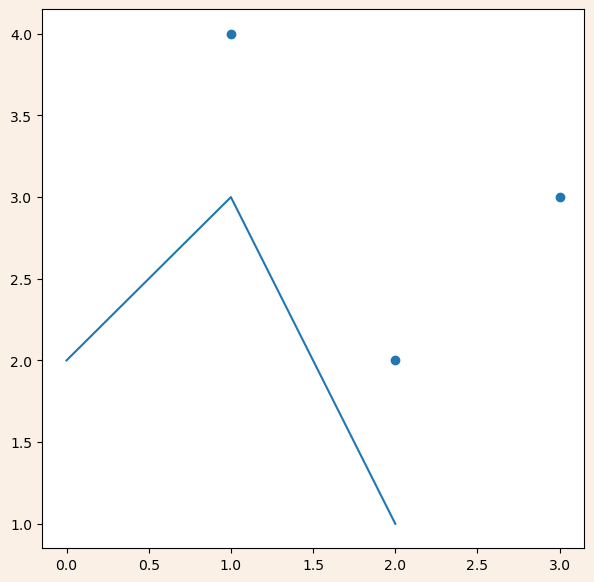
In [ ]:
figsize = (4, 4)
fig, ax = plt.subplots(figsize=figsize)
fig.suptitle('Title of a Figure')
fig.suptitle('Title font-size=30', fontsize=30)
fig.suptitle('Title font-family=monospace', fontfamily='monospace')
plt.show()
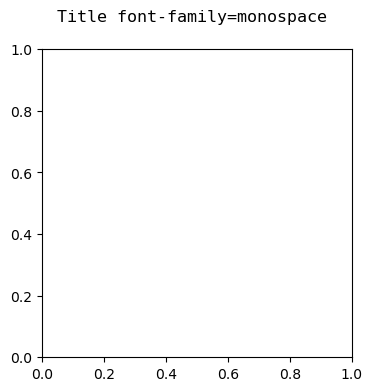
In [ ]:
fig, ax = plt.subplots(figsize=figsize)
ax.set_title('Title of a Ax')
ax.set_title('Title of a Ax font-size=30', fontsize=30)
ax.set_title('Title of a Ax font-family', fontfamily='monospace')
plt.show()
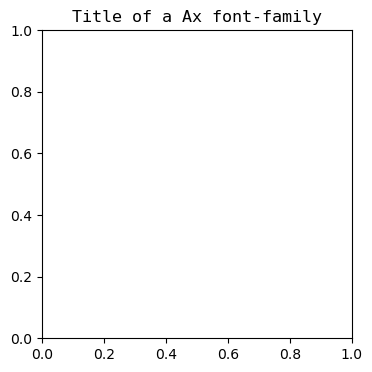
In [ ]:
fig, ax = plt.subplots(figsize=figsize)
ax.set_xlabel('X label', fontsize=20)
ax.set_ylabel('Y label', fontsize=20)
plt.show()

In [ ]:
fig, ax = plt.subplots(figsize=figsize)
fig.suptitle('Title font-family=monospace', color='darkblue', fontfamily='monospace', alpha=0.3)
ax.set_xlabel('X label', fontsize=20, color='darkblue', alpha=0.2)
ax.set_ylabel('Y label', fontsize=20, color='darkblue', alpha=0.2)
plt.show()
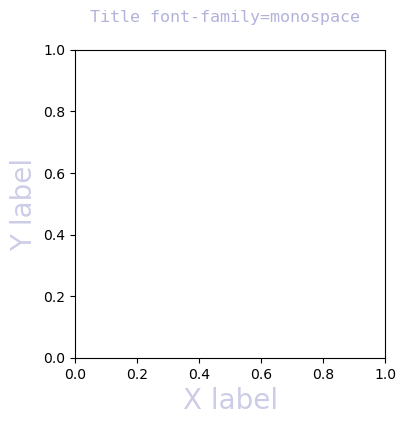
In [ ]:
fig, ax = plt.subplots(figsize=figsize)
fig = plt.figure(figsize=figsize)
ax1 = fig.add_subplot()
ax2 = ax1.twinx()
ax2.set_xlim(0, 100)
ax1.set_xlim(0, 100)
ax1.set_ylim(0, 100)
ax2.set_ylim(0, 0.1)
plt.show()
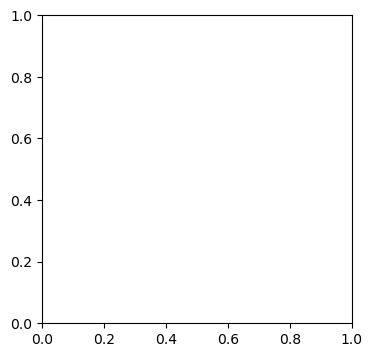
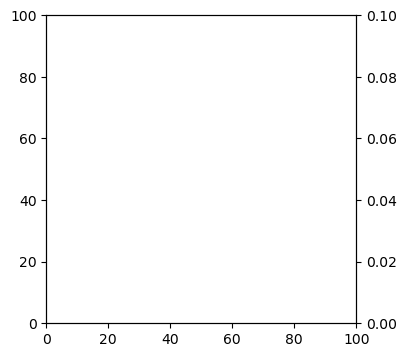
In [ ]:
fig, ax = plt.subplots(figsize=figsize)
ax1.set_title('Ax1')
ax1.set_ylabel('Data1', fontsize=30)
ax2.set_xlabel('Data2', fontsize=20)
fig.tight_layout()
plt.show()
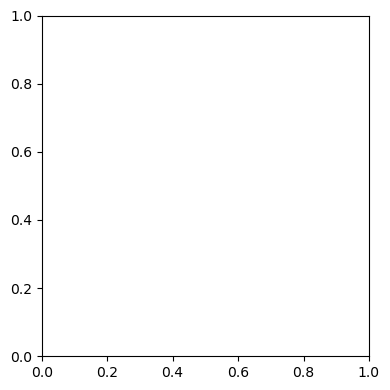
In [ ]:
tick_labelsize = 20
fig, ax = plt.subplots(figsize=figsize)
ax.tick_params(labelsize=tick_labelsize, length=10,
width=3,
bottom=False,
labelbottom=False,
top=True, labeltop=True,
left=True, labelleft=True,
right=True, labelright=True)
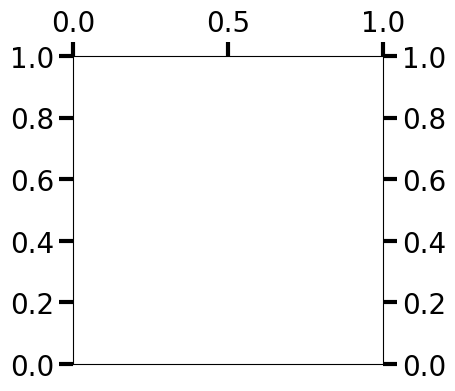
In [ ]:
fig, ax = plt.subplots(figsize=figsize)
ax.tick_params(labelsize=tick_labelsize,
length=10,
width=3,
bottom=False, labelbottom=False,
left=False, labelleft=False,
top=True, labeltop=True,
right=True, labelright=True)
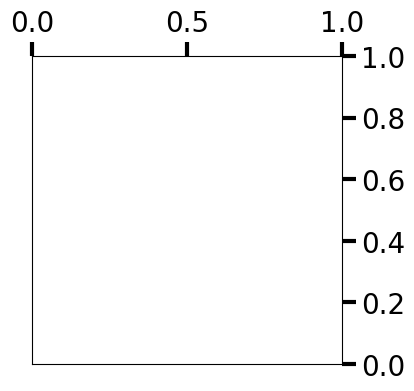
In [ ]:
# ratation 글자 기울기
fig, ax = plt.subplots(figsize=figsize)
ax.tick_params(labelsize=tick_labelsize,
length=10,
width=3,
rotation=30)
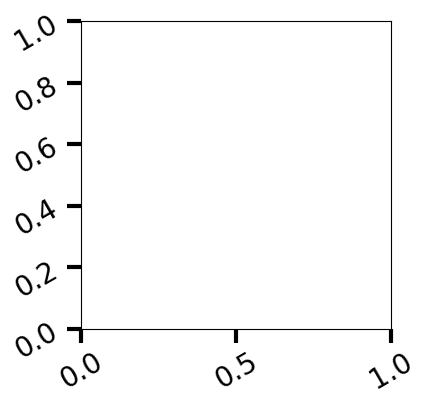
In [ ]:
fig, ax = plt.subplots(figsize=figsize)
ax.tick_params(axis='x',
labelsize=20, length=10, width=3, rotation=30)
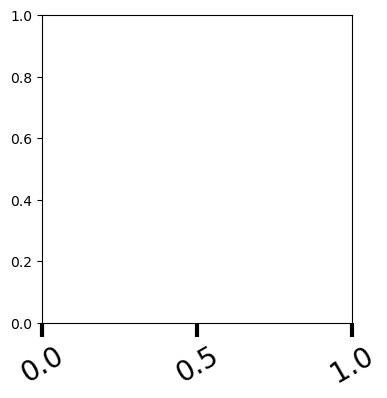
In [ ]:
fig, ax = plt.subplots(figsize=figsize)
ax.tick_params(axis='y',
labelsize=20,
length=10, width=3, rotation=30)
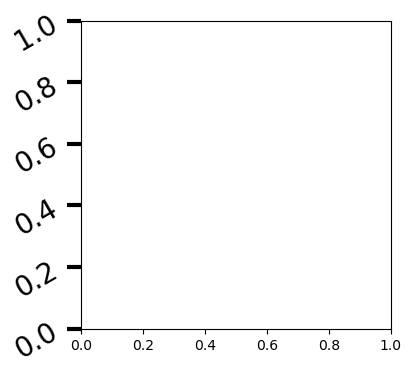
In [ ]:
fig, ax = plt.subplots(figsize=figsize)
ax.set_xlim(-1, 1)
ax.set_ylim(-1, 1)
ax.grid()
ax.tick_params(axis='both',
labelsize=15)
ax.text(0, 0,s='Hello', fontsize=30)
ax.text(0.5, 0,s='Hello2', fontsize=30)
Out[ ]:
Text(0.5, 0, 'Hello2')
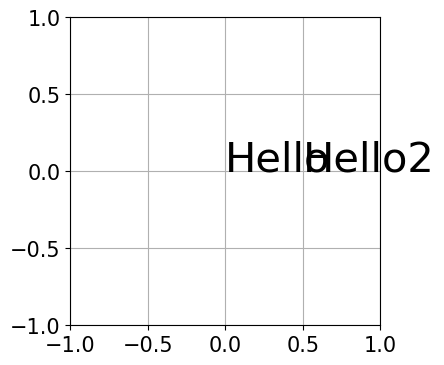
In [ ]:
fig, ax = plt.subplots(figsize=figsize)
ax.set_xlim(-1, 1)
ax.set_ylim(-1, 1)
ax.grid()
ax.text(0.0, 0.0,
va='center',
ha='left',
s="This is a test",
fontsize=12)
ax.text(0.0, 0.90,
va='center',
ha='center',
s="This is a test",
fontsize=12)
ax.text(0.05, 0.85,
va='center',
ha='right',
s="This is a test",
fontsize=12)
Out[ ]:
Text(0.05, 0.85, 'This is a test')
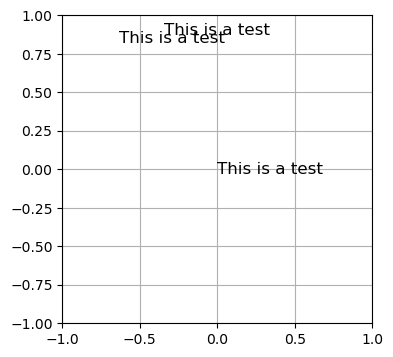
In [ ]:
fig, ax = plt.subplots(figsize=figsize)
ax.set_xlim(-1, 1)
ax.set_ylim(-1, 1)
ax.grid()
ax.text(0.0, 0.0,
va='top',
ha='left',
s="This is a test",
fontsize=12)
ax.text(0.0, 0.0,
va='top',
ha='right',
s="This is a test",
fontsize=12)
ax.text(0.0, 0.0,
va='bottom',
ha='left',
s="This is a test",
fontsize=12)
ax.text(0.0, 0.0,
va='bottom',
ha='right',
s="This is a test",
fontsize=12)
Out[ ]:
Text(0.0, 0.0, 'This is a test')
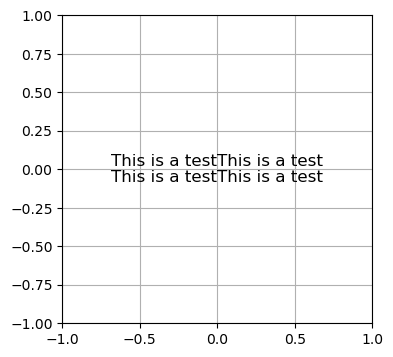
In [ ]:
fig, ax = plt.subplots(figsize=figsize)
ax.set_xlim(0, 101)
ax.set_ylim(0, 11)
ax.grid()
major_xticks = [i for i in range(0, 101, 20)]
minor_xticks = [i for i in range(0, 101, 5)]
ax.set_xticks(major_xticks)
ax.set_xticks(minor_xticks, minor=True)
ax.tick_params(axis='x',
labelsize=20,
length=10,
width=3,
rotation=30)
ax.tick_params(axis='x',
which='minor',
length=5,
width=2)
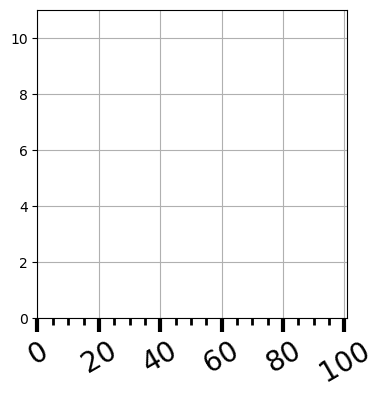
In [ ]:
fig, ax = plt.subplots(figsize=figsize)
ax.set_xlim(0, 101)
ax.set_ylim(0, 11)
ax.grid()
major_xticks = [i for i in range(0, 101, 20)]
minor_xticks = [i for i in range(0, 101, 5)]
major_yticks = [i for i in range(0, 11, 2)]
minor_yticks = [i for i in range(0, 11, 1)]
ax.set_xticks(major_xticks)
ax.set_xticks(minor_xticks, minor=True)
ax.set_yticks(major_yticks)
ax.set_yticks(minor_yticks, minor=True)
ax.tick_params(axis='x',
labelsize=20,
length=10,
width=3,
rotation=30)
ax.tick_params(axis='x',
which='minor',
length=5,
width=2)
ax.tick_params(axis='y',
labelsize=20,
length=10,
width=3,
rotation=30)
ax.tick_params(axis='y',
which='minor',
length=5,
width=2)
In [ ]:
import matplotlib.pyplot as plt
color_list = ['b', 'g', 'r', 'c', 'm', 'y']
fig, ax = plt.subplots(figsize=figsize)
ax.set_xlim(-1, 1)
ax.set_ylim(-1, len(color_list))
for c_idx, c in enumerate(color_list):
ax.text(x=0,
y=c_idx,
s="color="+c,
ha='center',
color=c)
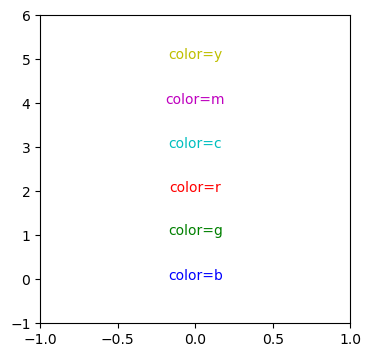
In [ ]:
import matplotlib.pyplot as plt
color_list = [(1., 0., 0.),
(0., 1., 0.),
(0., 0., 1.)]
fig, ax = plt.subplots(figsize=figsize)
ax.set_xlim(-1, 1)
ax.set_ylim(-1, len(color_list))
for c_idx, c in enumerate(color_list):
ax.text(x=0,
y=c_idx,
s=f"color={c}",
ha='center',
color=c)
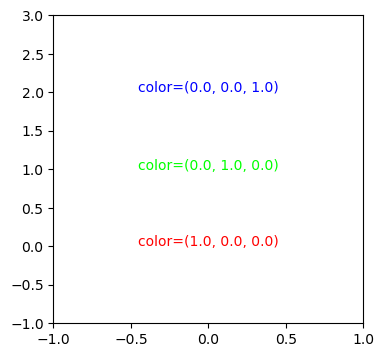
In [ ]:
import matplotlib.pyplot as plt
import numpy as np
np.random.seed(0)
n_data = 100
x_data = np.random.normal(0, 1, (n_data,))
y_data = np.random.normal(0, 1, (n_data,))
fig, ax = plt.subplots(figsize=figsize)
# 아래 두 방법 모두 scatter로 그린다
ax.scatter(x_data, y_data)
ax.plot(x_data, y_data, 'o')
Out[ ]:
[<matplotlib.lines.Line2D at 0x160984c90>]
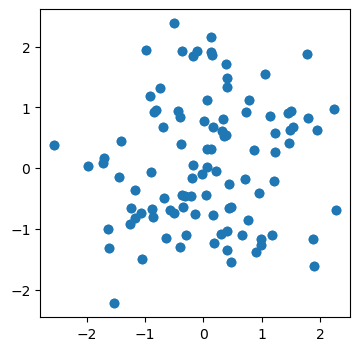
In [ ]:
import matplotlib.pyplot as plt
import numpy as np
np.random.seed(0)
n_data = 100
x_data = np.random.normal(0, 1, (n_data,))
y_data = np.random.normal(0, 1, (n_data,))
fig, ax = plt.subplots(figsize=figsize)
# 아래 두 방법 모두 scatter로 그린다
ax.scatter(x_data, y_data,
s=10,# markersize
color='r',)
ax.plot(x_data, y_data, 'o',
color='red',
markersize=10)
Out[ ]:
[<matplotlib.lines.Line2D at 0x160a7ae90>]
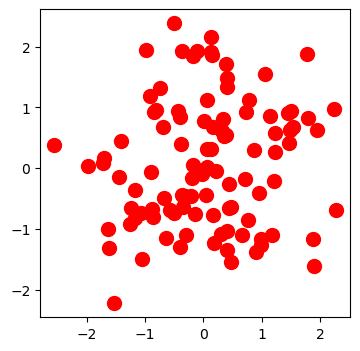
In [ ]:
import matplotlib.pyplot as plt
import numpy as np
np.random.seed(0)
x_min, x_max = -5, 5
n_data = 300
x_data = np.random.uniform(x_min, x_max, n_data)
y_data = x_data + -0.5*np.random.normal(0, 1, n_data)
pred_x = np.linspace(x_min, x_max, 10) # 임이의 노이즈 생성
pred_y = pred_x
fig, ax = plt.subplots(figsize=figsize)
ax.scatter(x_data, y_data,s=1) # scatter
ax.plot(pred_x, pred_y,
color='r',
linewidth=1) # line
Out[ ]:
[<matplotlib.lines.Line2D at 0x160aad590>]

In [ ]:
import matplotlib.pyplot as plt
import numpy as np
n_data = 10
x_data = np.linspace(0, 10, n_data)
y_data = np.linspace(0, 10, n_data)
s_arr = np.linspace(10, 500, n_data)
fig, ax = plt.subplots(figsize=figsize)
ax.scatter(x_data, y_data, s=s_arr)
Out[ ]:
<matplotlib.collections.PathCollection at 0x160c174d0>
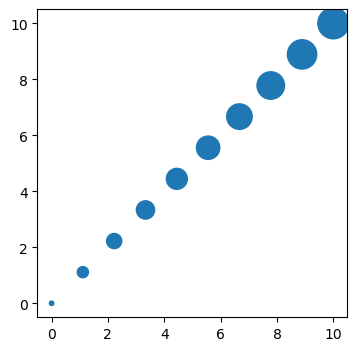
In [ ]:
import matplotlib.pyplot as plt
import numpy as np
n_data = 10
x_data = np.linspace(0, 10, n_data)
y_data = np.linspace(0, 10, n_data)
c_arr = [(c/n_data, c/n_data, c/n_data) for c in range(n_data)]
fig, ax = plt.subplots(figsize=figsize)
ax.scatter(x_data, y_data, c=c_arr, s=s_arr)
Out[ ]:
<matplotlib.collections.PathCollection at 0x160c83c50>
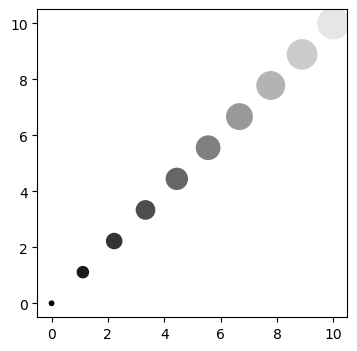
In [ ]:
import matplotlib.pyplot as plt
import numpy as np
np.random.seed(0)
n_data = 500
x_data = np.random.normal(0, 1, (n_data,))
y_data = np.random.normal(0, 1, (n_data,))
s_arr = np.random.uniform(100, 500, n_data)
c_arr = [np.random.uniform(0, 1, 3) for _ in range(n_data)]
fig, ax = plt.subplots(figsize=figsize)
ax.scatter(x_data, y_data, c=c_arr, s=s_arr)
ax.scatter(x_data, y_data, c=c_arr, s=s_arr, alpha=0.1)
Out[ ]:
<matplotlib.collections.PathCollection at 0x160865990>
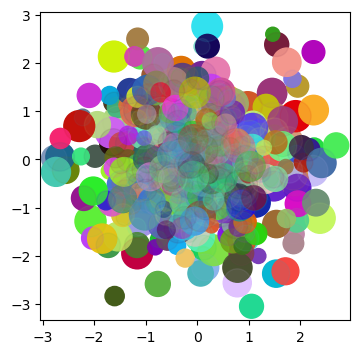
'새싹 > TIL' 카테고리의 다른 글
[핀테커스] 230920 python 수학 실습 (0) | 2023.09.20 |
---|---|
[핀테커스] 230919 python 수학 실습 (1) | 2023.09.19 |
[핀테커스] 230915 미니프로젝트 (0) | 2023.09.15 |
[핀테커스] 230914 데이터 시각화 (3) | 2023.09.14 |
[핀테커스] 230913 pandas merge & metplot 시각화 (0) | 2023.09.13 |